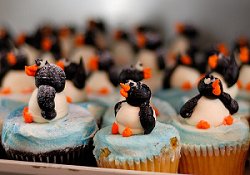
Photo By mkrigsman
If you’re a developer, you probably find yourself using Linux at least some of the time. Knowing your way around the OS can make your life a lot easier, and I’ve sometimes found myself wishing I knew certain commands or concepts a lot sooner. I’m no expert, but since I thought it might be useful, I’ve put together a short quiz to test your Linux skills.
So, let’s get started…
- What does the cd command do when handed each of the following inputs: “-“, “/tmp” and “~”? [+]
- “cd -” changes the current directory to the directory you were previously in.
- “cd /tmp” changes the current directory to “/tmp”
- “cd ~” changes the current directory to your home directory.
- What are two ways of copying a text file without using the cp command? [+]
- cat the file and redirect the output to a new file.
- Use the scp command (secure copy).
- You want your console window to continuously show you the latest updates to a log file as its being updated. How would you do this? [+]
- Use the tail command with the -f flag.
- Use the less command with the +F option (personal favorite).
- What is the difference between the “which” and “whereis” commands? [+]
- which shows the full path of a given command.
- whereis locates source, binary, and manual files for a given command.
- How would you find all of the files modified within the last day under a particular directory structure? [+]
- find ./ -type f -mtime -1
- What is the purpose of the “/etc” and “/var” directories? [+]
- The main directory layout for all Linux systems is based on the Filesystem Hierarchy Standard (FHS).
- The “/etc” directory contains host-specific, system-wide configuration files (sometimes called “Editable Text Configuration”).
- The “/var” directory contains “variable files”, or files whose content is expected to continuously change during the normal operation of the system (ex: log files).
- What are “cron jobs”? [+]
- Linux systems have a program called “cron” which runs in the background and executes jobs (commands, scripts, etc) based on a schedule defined in a crontab file. This allows you to routinely do certain tasks. A “cron job” refers to a job run by the cron daemon.
- What does the “nohup” command do? [+]
- Short for No Hang Up, this command allow you to execute a command that will keep going after you’ve logged out (ie, one that ignores hang up signals).
- You have a directory tree which contains all of the files for a web application. You want to make a list of all of the files that contain a phone number in the format of “(XXX) XXX-XXXX”. How would you do this? [+]
- The key insight is to use the grep command with the -R flag (recursive directory search), -l flag (list files) and a regular expression to find the phone numbers.
- As a side note, this used to be a popular interview question that Amazon gave to potential applicants (for more info, see the “Notes” section at the bottom of this post).
- What is the name of the official Linux kernel mascot? [+]
- Tux.
Mouse over or click the +’s to see possible answers (though I didn’t include all possible answers – so you may have thought of some solutions that aren’t listed).
If you found yourself doing poorly, or just want to refresh your linux skill set, check out:
http://www.funtoo.org/wiki/Linux_Fundamentals,_Part_1
They have some of the best tutorials I’ve seen on Linux. However, if anyone knows of any other good tutorials let me know and I’ll add them here too.
Notes:
- For more information on question 9, click here and go to the section titled “Area Number Three: Scripting and Regular Expressions”.